- import mysql.connector
- from mysql.connector import Error
-
- try:
- # 連接 MySQL/MariaDB 資料庫
- connection = mysql.connector.connect(
- host='localhost', # 主機名稱
- database='officeguide_db', # 資料庫名稱
- user='officeguide', # 帳號
- password='your_password') # 密碼
-
- if connection.is_connected():
-
- # 顯示資料庫版本
- db_Info = connection.get_server_info()
- print("資料庫版本:", db_Info)
-
- # 顯示今朝利用的資料庫
- cursor = connection.cursor()
- cursor.execute("SELECT DATABASE();")
- record = cursor.fetchone()
- print("今朝利用的資料庫:", record)
-
- except Error as e:
- print("資料庫毗連失敗:", e)
-
- finally:
- if (connection.is_connected()):
- cursor.close()
- connection.close()
- print("資料庫連線已封閉")
複製代碼
- import mysql.connector
- from mysql.connector import Error
-
- try:
- # 毗鄰 MySQL/MariaDB 資料庫
- connection = mysql.connector.connect(
- host='localhost', # 主機名稱
- database='officeguide_db', # 資料庫名稱
- user='officeguide', # 帳號
- password='your_password') # 密碼
-
- # 查詢資料庫
- cursor = connection.cursor()
- cursor.execute("SELECT name, age FROM persons;")
-
- # 取回掃數的資料
- records = cursor.fetchall()
- print("資料筆數:", cursor.rowcount)
-
- # 列出查詢的資料
- for (name, age) in records:
- print("Name: %s, Age: %d" % (name, age))
-
- except Error as e:
- print("資料庫毗連失敗:", e)
-
- finally:
- if (connection.is_connected()):
- cursor.close()
- connection.close()
- print("資料庫連線已封閉")
複製代碼
安裝 Python 的 MySQL Connector 模組
安裝 Python 的 MySQL Connector 模組
安裝好以後,就能夠起頭使用了 Python 的 MySQL Connector 模組。
毗連資料庫
以下是利用 MySQL Connector 模組連接資料庫的範例:
- import mysql.connector
- from mysql.connector import Error
-
- try:
- # 毗鄰 MySQL/MariaDB 資料庫
- connection = mysql.connector.connect(
- host='localhost', # 主機名稱
- database='officeguide_db', # 資料庫名稱
- user='officeguide', # 帳號
- password='your_password') # 暗碼
-
- # 更新資料
- sql = "DELETE FROM persons WHERE id = %s;"
- cursor = connection.cursor()
- cursor.execute(sql, (6,))
-
- # 確認資料有存入資料庫
- connection.commit()
-
- except Error as e:
- print("資料庫毗鄰失敗:", e)
-
- finally:
- if (connection.is_connected()):
- cursor.close()
- connection.close()
複製代碼
- import mysql.connector
- from mysql.connector import Error
-
- try:
- # 毗鄰 MySQL/MariaDB 資料庫
- connection = mysql.connector.connect(
- host='localhost', # 主機名稱
- database='officeguide_db', # 資料庫名稱
- user='officeguide', # 帳號
- password='your_password') # 密碼
-
- # 新增資料
- sql = "INSERT INTO persons (name, age, city) VALUES (%s, %s, %s);"
- new_data = ("Jack", 13, "Kaohsiung")
- cursor = connection.cursor()
- cursor.execute(sql, new_data)
-
- # 確認資料有存入資料庫
- connection.commit()
-
- except Error as e:
- print("資料庫毗連失敗:", e)
-
- finally:
- if (connection.is_connected()):
- cursor.close()
- connection.close()
複製代碼
參考資料:MySQL 官方文件、pynative
https://officeguide.cc/python-mysql-mariadb-database-connector-tutorial-examples/
介紹若何利用 Python 的 MySQL Connector 模組毗連 MySQL/MariaDB 資料庫,進行查詢、新增或刪除等各類操作。
Python 有很多 MySQL/MariaDB 資料庫相關的模組,而最常被利用的就是 MySQL Connector 與 MySQLdb 這兩個模組,以下是 MySQL Connector 模組的利用體例。
安裝 MySQL Connector 模組
開啟 Windows 中的命令提醒自元,利用 pip 安裝 Python 的 MySQL Connector 模組:
- import mysql.connector
- from mysql.connector import Error
-
- try:
- # 連接 MySQL/MariaDB 資料庫
- connection = mysql.connector.connect(
- host='localhost', # 主機名稱
- database='officeguide_db', # 資料庫名稱
- user='officeguide', # 帳號
- password='your_password') # 密碼
-
- # 查詢資料庫
- cursor = connection.cursor()
- cursor.execute("SELECT name, age FROM persons;")
-
- # 列出查詢的資料
- for (name, age) in cursor:
- print("Name: %s, Age: %d" % (name, age))
-
-
- except Error as e:
- print("資料庫毗鄰失敗:", e)
-
- finally:
- if (connection.is_connected()):
- cursor.close()
- connection.close()
- print("資料庫連線已關閉")
複製代碼
網頁設計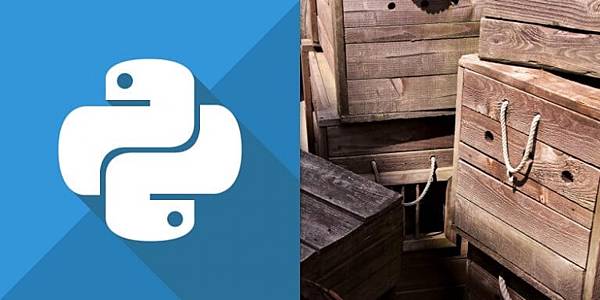
點竄資料
以下是利用 UPDATE 更新資料的範例:
資料筆數: 4
Name: Arden, Age: 32
Name: Bond, Age: 54
Name: Cole, Age: 12
Name: Dana, Age: 19
資料庫連線已封閉
新增資料
以下是利用 INSERT 新增資料的典範榜樣:
- import mysql.connector
- from mysql.connector import Error
-
- try:
- # 連接 MySQL/MariaDB 資料庫
- connection = mysql.connector.connect(
- host='localhost', # 主機名稱
- database='officeguide_db', # 資料庫名稱
- user='officeguide', # 帳號
- password='your_password') # 暗碼
-
- # 更新資料
- sql = "UPDATE persons SET age = %s WHERE id = %s;"
- cursor = connection.cursor()
- cursor.execute(sql, (27, 6))
-
- # 確認資料有存入資料庫
- connection.commit()
-
- except Error as e:
- print("資料庫連接失敗:", e)
-
- finally:
- if (connection.is_connected()):
- cursor.close()
- connection.close()
複製代碼
網頁設計
Name: Arden, Age: 32
Name: Bond, Age: 54
Name: Cole, Age: 12
Name: Dana, Age: 19資料庫連線已封閉
也能夠使用 fetchall 將資料一次掃數抓到 Python 中再處理:
- pip install mysql-connector-python
複製代碼
刪除資料
以下是使用 DELETE 刪除資料的規範:
資料庫版本: 5.5.5-10.4.8-MariaDB
今朝使用的資料庫: ('officeguide_db',)
資料庫連線已封閉
查詢資料
以下是利用 SELECT 查詢資料的規範:
文章來自: